Data Grid - Getting started
Get started with the last React data grid you will need. Install the package, configure the columns, provide rows, and you are set.
Installation
Using your favorite package manager, install @mui/x-data-grid-pro
for the full-featured enterprise grid, or @mui/x-data-grid
for the free community version.
// with npm
npm install @mui/x-data-grid
// with yarn
yarn add @mui/x-data-grid
The grid has a peer dependency on one MUI component. If you are not already using MUI in your project, you can install it with:
// with npm
npm install @mui/material
// with yarn
yarn add @mui/material
Quickstart
First, you have to import the component as below.
To avoid name conflicts the component is named DataGridPro
for the full-featured enterprise grid, and DataGrid
for the free community version.
import { DataGrid } from '@mui/x-data-grid';
Define rows
Rows are key-value pair objects, mapping column names as keys with their values.
You should also provide an id
property on each row to allow delta updates and better performance.
Here is an example
const rows: GridRowsProp = [
{ id: 1, col1: 'Hello', col2: 'World' },
{ id: 2, col1: 'DataGridPro', col2: 'is Awesome' },
{ id: 3, col1: 'MUI', col2: 'is Amazing' },
];
Define columns
Comparable to rows, columns are objects defined with a set of attributes of the GridColDef
interface.
They are mapped to the rows through their field
property.
const columns: GridColDef[] = [
{ field: 'col1', headerName: 'Column 1', width: 150 },
{ field: 'col2', headerName: 'Column 2', width: 150 },
];
You can import GridColDef
to see all column properties.
Demo
Putting it together, this is all you need to get started, as you can see in this live and interactive demo:
import React from 'react';
import { DataGrid, GridRowsProp, GridColDef } from '@mui/x-data-grid';
const rows: GridRowsProp = [
{ id: 1, col1: 'Hello', col2: 'World' },
{ id: 2, col1: 'DataGridPro', col2: 'is Awesome' },
{ id: 3, col1: 'MUI', col2: 'is Amazing' },
];
const columns: GridColDef[] = [
{ field: 'col1', headerName: 'Column 1', width: 150 },
{ field: 'col2', headerName: 'Column 2', width: 150 },
];
export default function App() {
return (
<div style={{ height: 300, width: '100%' }}>
<DataGrid rows={rows} columns={columns} />
</div>
);
}
TypeScript
In order to benefit from the CSS overrides and default prop customization with the theme, TypeScript users need to import the following types. Internally, it uses module augmentation to extend the default theme structure.
// When using TypeScript 4.x and above
import type {} from '@mui/x-data-grid/themeAugmentation';
import type {} from '@mui/x-data-grid-pro/themeAugmentation';
const theme = createTheme({
components: {
// Use `MuiDataGrid` on both DataGrid and DataGridPro
MuiDataGrid: {
styleOverrides: {
root: {
backgroundColor: 'red',
},
},
},
},
});
Licenses
While MUI Core is entirely licensed under MIT, MUI X serves a part of its components under a commercial license. Please pay attention to the license.
Plans
The component comes in different plans:
Community: It's MIT license. It's free forever and available on npm as
@mui/x-data-grid
. It currently includes the DataGrid component.Pro and Premium: It's a commercial license. It's available on npm as
@mui/x-data-grid-pro
and includes the DataGridPro component. Features only available in the commercial version are suffixed in the documentation with the following marks:: For features exclusive to Pro and Premium plans.
: For features exclusive to the Premium plan.
You can check the feature comparison for more details. See Pricing for details on purchasing licenses.
MIT vs. commercial
How do we decide if a feature is MIT or commercial?
We have been building MIT React components since 2014, and have learned much about the strengths and weaknesses of the MIT license model. The health of this model is improving every day. As the community grows, it increases the probability that developers contribute improvements to the project. However, we believe that we have reached the sustainability limits of what the model can support for advancing our mission forward. We have seen too many MIT licensed components moving slowly or getting abandoned. The community isn't contributing improvements as fast as the problems deserved to be solved.
We are using a commercial license to forward the development of the most advanced features, where the MIT model can't sustain it. A solution to a problem should only be commercial if it has no MIT alternatives.
We provide three plans:
- Community. This plan contains the MIT components that are sustainable by the contributions of the open-source community. Free forever.
- Pro. This plan contains the features that are at the limit of what the open-source model can sustain. For instance, providing a very comprehensive set of components. From a price perspective, the plan is designed to be accessible to most professionals.
- Premium. This plan contains the most advanced features.
Feature comparison
The following table summarizes the features available in the community DataGrid
and enterprise DataGridPro
components.
All the features of the community version are available in the enterprise one.
The enterprise components come in two plans: Pro and Premium.
Features | Community | Pro | Premium |
---|---|---|---|
Column | |||
Column groups | ๐ง | ๐ง | ๐ง |
Column spanning | ๐ง | ๐ง | ๐ง |
Column resizing | โ | โ | โ |
Column reorder | โ | โ | โ |
Column pinning | โ | โ | โ |
Row | |||
Row height | โ | โ | โ |
Row spanning | ๐ง | ๐ง | ๐ง |
Row reordering | โ | ๐ง | ๐ง |
Row pinning | โ | ๐ง | ๐ง |
Selection | |||
Single row selection | โ | โ | โ |
Checkbox selection | โ | โ | โ |
Multiple row selection | โ | โ | โ |
Cell range selection | โ | โ | ๐ง |
Filtering | |||
Quick filter | ๐ง | ๐ง | ๐ง |
Column filters | โ | โ | โ |
Multi-column filtering | โ | โ | โ |
Sorting | |||
Column sorting | โ | โ | โ |
Multi-column sorting | โ | โ | โ |
Pagination | |||
Pagination | โ | โ | โ |
Pagination > 100 rows per page | โ | โ | โ |
Editing | |||
Row editing | โ | โ | โ |
Cell editing | โ | โ | โ |
Import & export | |||
CSV export | โ | โ | โ |
โ | โ | โ | |
Clipboard | โ | ๐ง | ๐ง |
Excel export | โ | โ | ๐ง |
Rendering | |||
Customizable components | โ | โ | โ |
Column virtualization | โ | โ | โ |
Row virtualization > 100 rows | โ | โ | โ |
Group & Pivot | |||
Tree data | โ | โ | โ |
Master detail | โ | โ | โ |
Grouping | โ | โ | ๐ง |
Aggregation | โ | โ | ๐ง |
Pivoting | โ | โ | ๐ง |
Misc | |||
Accessibility | โ | โ | โ |
Keyboard navigation | โ | โ | โ |
Localization | โ | โ | โ |
Evaluation (trial) licenses
You are free to install and try DataGridPro
as long as it is not used for the development of a feature intended for production.
Please take the component for a test run, no need to contact us.
License key
For commercially licensed software, a license key is provided. This removes the watermark and console warning when valid. This license key is meant as a reminder for developers and their team to know when they forgot to license the software or renew the license.
License key installation
Once you purchase a license, you'll receive a license key by email. This key should be installed to remove the watermark and console warnings. You must set the license key before rendering the first component. You only need to install the key once in your application.
import { LicenseInfo } from '@mui/x-data-grid-pro';
LicenseInfo.setLicenseKey(
'x0jTPl0USVkVZV0SsMjM1kDNyADM5cjM2ETPZJVSQhVRsIDN0YTM6IVREJ1T0b9586ef25c9853decfa7709eee27a1e',
);
Security
The check of the license key is done without making any network requests.
The license key is designed to be public, the only thing we ask of licensed users is to not proactively publicize their license key. Exposing the license key in a JavaScript bundle is expected.
Validation errors
If the validation of the license key fails, the component displays a watermark and provides a console warning in both development and production. End users can still use the component.
Here are the different possible validation errors:
Missing license key
If the license key is missing, the component will look something like this:
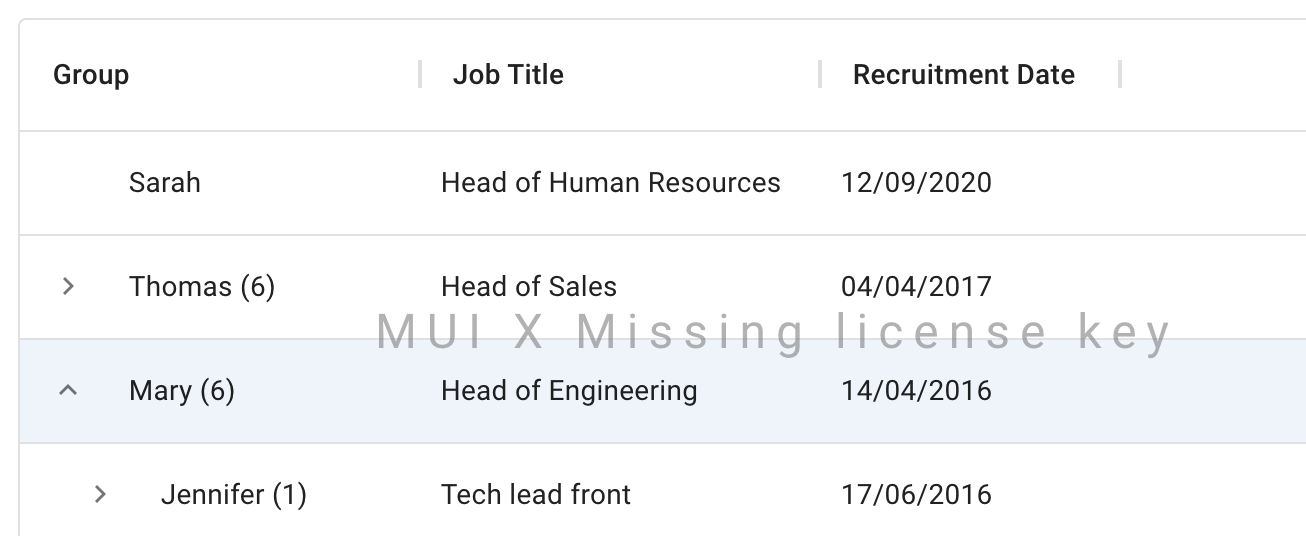
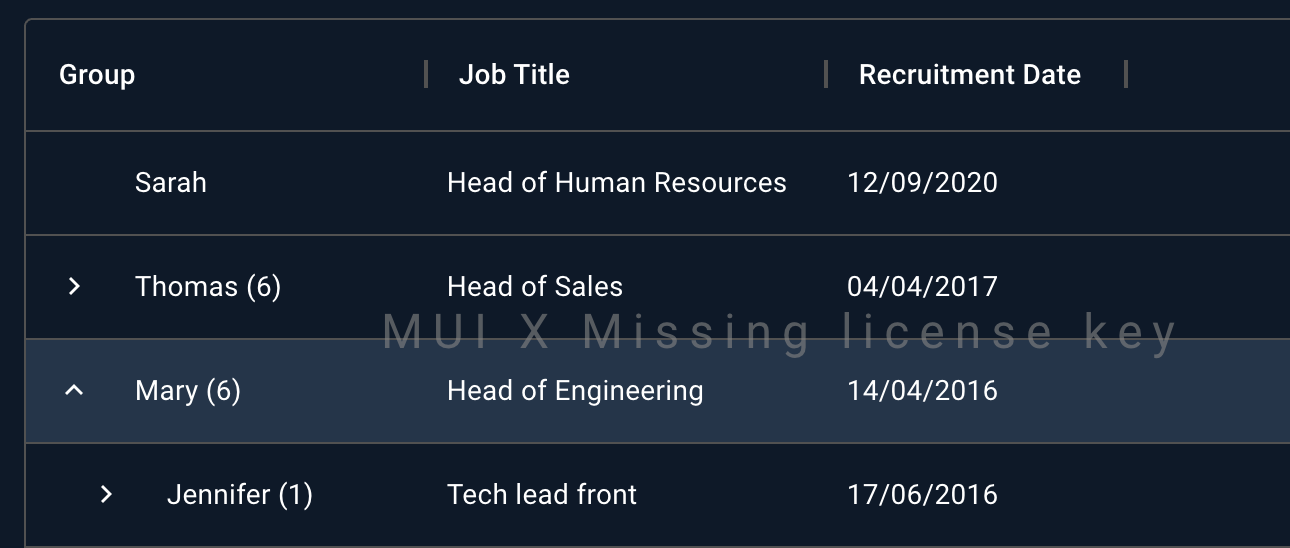
Note that you are still allowed to use the component for evaluation purposes in this case.
License key expired
The licenses are perpetual, the license key will work forever with the current version of the software.
However, access to updates/upgrades is not perpetual. Installing a version of the component released after the license key has expired will trigger a watermark and console message. For example, if you purchase a one-year license today, you are not licensed to install a version released two years in the future, but you can optionally update to any version, including major versions, if it's released in the next 12 months.
Invalid license key
The license key you have installed is not as issued by MUI.
Support
GitHub
We use GitHub issues as a bug and feature request tracker. If you think you have found a bug, or have a new feature idea, please start by making sure it hasn't already been reported or fixed. You can search through existing issues and pull requests to see if someone has reported one similar to yours.
StackOverflow
For crowdsourced answers from expert MUI developers in our community. StackOverflow is also visited from time to time by the maintainers of MUI.
Professional support
When purchasing an MUI X Pro or Premium license you get access to professional support for a limited duration. Support is available on multiple channels, but the recommended channel is GitHub issues. If you need to share private information, you can also use email.
- MUI X Pro: No SLA is provided but MUI's maintainers give these issues more attention than the ones from the Community plan. The channels:
- GitHub: Open a new issue and leave your Order ID.
- Email (only to share private information): Open a new issue or send an email at x@mui.com.
- MUI X Premium: Same as MUI X Pro, but with priority over Pro, and a 48 hour SLA for the first answer.
- GitHub: this plan is not available yet
- Emails: this plan is not available yet
- MUI X Premium Priority: Same as MUI X Premium but with a 24 hours SLA for the first answer.
- GitHub: this plan is not available yet
- Emails: this plan is not available yet
Roadmap
Here is the public roadmap. It's organized by quarter.
โ ๏ธ Disclaimer: We operate in a dynamic environment, and things are subject to change. The information provided is intended to outline the general framework direction, for informational purposes only. We may decide to add or remove new items at any time, depending on our capability to deliver while meeting our quality standards. The development, releases, and timing of any features or functionality remains at the sole discretion of MUI. The roadmap does not represent a commitment, obligation, or promise to deliver at any time.